PHP Interview Program
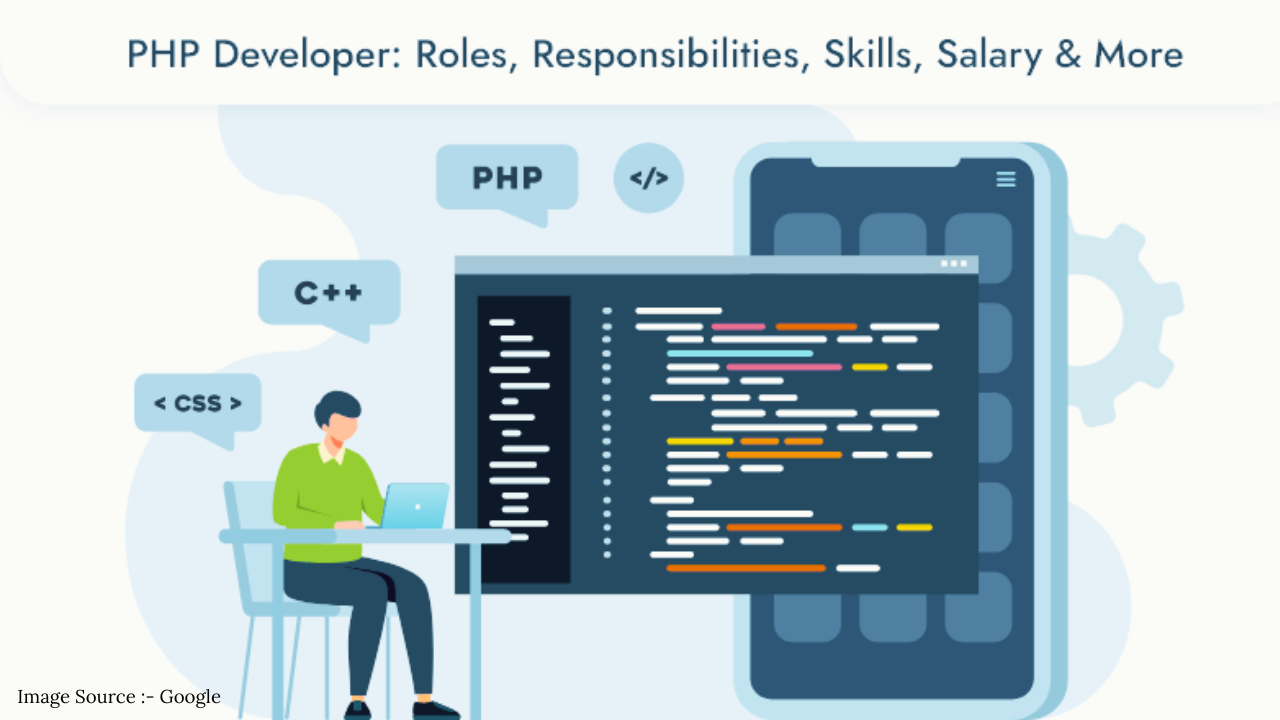
1. write a program for Fibonacci Sequence.
2. write a program for Palindrome Check.
3. write a program for calculate the factorial of a number.
4. write a program for Reverse a String.
5. write a program for Check Prime Number .
6. write a program for Sum of Digits.
7. How to find the Largest Element in an Array.
8. write a program for Remove Duplicates value from an Array.
9. write a program for Merge and Sort Arrays.
10. write a program for Count the Occurrences of Each Element in an Array.
1. write a program for Fibonacci Sequence.
Here ,We are writing fibonacci sequence code with complete example.
<?php
function fibonacci($n) {
$fib = [0, 1];
for ($i = 2; $i < $n; $i++) {
$fib[$i] = $fib[$i - 1] + $fib[$i - 2];
}
return $fib;
}
echo "<pre>";
// Example usage 10 you can tale any number like 10,20, 30,50 etc.
print_r(fibonacci(10));
?>

2. write a program for Palindrome Check if a string is a palindrome.
<?php
function isPalindrome($string) {
$cleanedString = preg_replace('/[^a-zA-Z0-9]/', '', strtolower($string));
return $cleanedString === strrev($cleanedString);
}
// Example usage
echo isPalindrome('A man, a plan, a canal, Panama'); // true output is 1.
?>
3. write a program for calculate the factorial of a number.
<?php
function factorial($n) {
if ($n === 0) {
return 1;
}
return $n * factorial($n - 1);
}
// Example usage
echo factorial(5);
// if you are execute this program then out put is 120.
?>
4. Write a PHP function to reverse a string.
<?php
function reverseString($str) {
return strrev($str);
}
// Example usage
echo reverseString('hello welcom to my website');
echo "<br>";
// 2 example with simple variable.
$reverse =" renoitidnoc ria kaj ot emoclew";
echo strrev($reverse);
?>
An example of this code is given below
5. write a program for Check Prime Number.
<?php
function isPrime($num) {
if ($num <= 1) {
return false;
}
for ($i = 2; $i <= sqrt($num); $i++) {
if ($num % $i == 0) {
return false;
}
}
return true;
}
// Example usage
echo isPrime(97); // true
?>
An example of this code is given below
out put is 1. if condition true /false otherwise output is not show.
6. write a program for Sum of Digits.
<?php
function sumOfDigits($num) {
$sum = 0;
while ($num > 0) {
$sum += $num % 10;
$num = (int)($num / 10);
}
return $sum;
}
// Example usage
echo sumOfDigits(123); // output is 6
echo "</br>";
//simple example od sum of digites.
$sum1 = 100;
$sum2 = 400;
$sum3 = $sum1 + $sum2;
echo "$sum3";
// output is 500
?>
7. How to find the Largest Element in an Array.
<?php
function largestElement($arr) {
return max($arr);
}
// Example usage
echo largestElement([1, 2, 3, 4, 5,100]); // output of this program is 100.
// simple example of array
$array =array(1,2,3,4,5,6,7,8,9,10);
echo max($array); // output of this program is 10.
?>
The Billion Dollar Abode: Inside Mukesh Ambani House | Mukesh Ambani Net Worth
8. write a program for Remove Duplicates value from an Array.
<?php
function removeDuplicates($array) {
return array_values(array_unique($array));
}
// Example usage
print_r(removeDuplicates([1, 2, 2, 3, 4, 4, 5,10,10,12,12,]));
?>
An example of this code is given below

9. write a program for Merge and Sort Arrays.
<?php
function mergeAndSort($arr1, $arr2) {
$merged = array_merge($arr1, $arr2);
sort($merged);
return $merged;
}
// Example usage
print_r(mergeAndSort([3, 2, 1], [6, 5, 4]));
?>
An example of this code is given below

10. write a program for Count the Occurrences of Each Element in an Array.
<?php
function countOccurrences($arr) {
return array_count_values($arr);
}
// Example usage
print_r(countOccurrences(['apple', 'banana', 'apple', 'orange', 'banana', 'banana']));
?>
An example of this code is given below

Cast Certificate online apply free
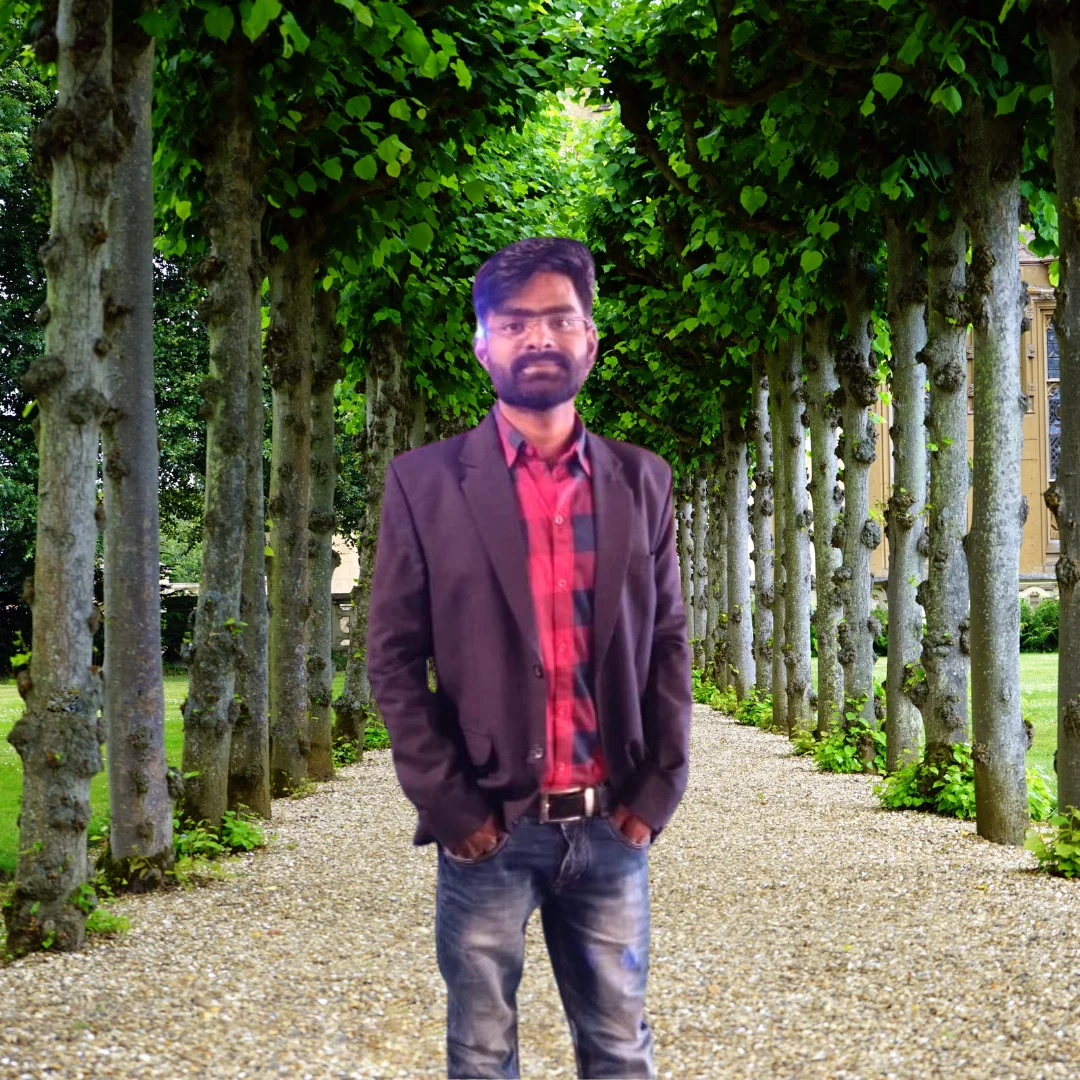
मैं एक कंप्यूटर इंजीनियर हूं और मुझे आर्टिकल लिखने का 3 साल का अनुभव है मैं टेक्नॉल्जी और एजुकेशन से जुडी पोस्ट Dailysearchs.com पर डालता हूं। धन्यवाद